Converting alphabet letters to numbers is common in computer science and programming. This process, known as letter-to-number mapping, involves assigning a numerical value to each letter of the alphabet. Python can accomplish this through various methods, including dictionaries, ASCII values, and built-in functions.
Whether you are a beginner or an experienced programmer, this guide will provide you with a comprehensive understanding of how to convert alphabet letters to numbers using Python. With step-by-step instructions and clear explanations, you will be able to apply this knowledge to solve real-world problems and enhance your coding skills.
Converting alphabet letters to numbers was used as the earliest means of encryption of the English alphabet to a corresponding pre-assigned numerical value. Its roots can be traced back to Julius Caesar, who introduced the Caesar cipher during times of war. The Caesar cipher assigned a number to each letter in the alphabet. The encryption was done by subtracting the number by two and replacing the original letter with the letter corresponding to the resultant number. Caesar cipher was a huge success in the old times and was used to relay messages throughout the war, but converting alphabet letters to numbers is out of fashion in the world with modern technology.
Still, it provides a small coding exercise, and this article will show three different methods to do the same. The corresponding number to the letter is called it’s ordinal. American Standard Code for Information Interchange (ASCII) provides an ordinal number to every character available in its language. All letters of the English alphabet are also assigned ordinal numbers, which can be used.
The numbering scheme used in this article for each letter is shown in the table below:
Character | a | b | c | d | e | f | g | h | i | j | k | l | m | n | o | p | q | r | s | t | u | v | w | x | y | z | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
Assigned Ordinal | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | 27 | 28 | 29 | 30 | 31 | 32 | 33 | 34 | 35 | 36 | 37 | 38 | 39 | 40 | 41 | 42 | 43 | 44 | 45 | 46 | 47 | 48 | 49 | 50 | 51 | 52 |
It should be noted that the lower case, as well as the upper case letters, are being considered in this article. The article provides three different methods to convert alphabet letters to numbers. They are as follows:
- Using two separate lists
- Using a dictionary iterable
- Using ord() function
Method 1: Using Two Separate Lists
In this method, two different lists of equal size are maintained. The first list is a list of all letters of the English alphabet, in both lower case followed by upper case. The second list contains numbers from 1 to 52, each number assigned to its corresponding letter. On reading the input data, each character from the input data is checked for its presence in the letters list; if it is found, its index in the list is found. It is replaced by the element from the numbers list present at the same index. It also replaces a space with a white tab to produce cleaner output. It is represented in the code below:
charstr='abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
chars=list(charstr)
nums=[str(i) for i in range(1,53)]
with open('data.txt','r') as f:
data=f.read()
output=''
for i in range(len(data)):
if data[i] in chars:
pos=chars.index(data[i])
output=output+nums[pos]+' '
elif data[i]==' ':
output=output+'\t'
else:
output=output+data[i]+' '
print('Output of Two Lists: '+output)
The charstr in the above code is a string of all letters of the English Alphabet in lower case followed by letters in upper case. The list() function of Python helps in converting the given string to a list of all the characters that form the string. nums is another list that contains the string form of all the numbers from 1 to 52. It should be noted that the range for loop mentions 1 to 53 as the for loop runs from the first input mentioned to the second input mentioned but does not include the second input. The program here uses a Text file called ‘data.txt’ to take its input which needs to be encoded. The data.txt file contains the following text:
Hello everyone, How are you doing?
Looks something fishy? Well it is!!

The code iterates through all the contents of the above-given text file and if it finds a letter, it replaces it with the corresponding element from nums list. The index() function of the Python list provides the letter’s position in the chars list. It lets the other characters as it is. But in place of space, it leaves a white tab. The output is as shown:
Output by Two Lists : 34 5 12 12 15 5 22 5 18 25 15 14 5 , 34 15 23 1 18 5 25 15 21 4 15 9 14 7 ?
38 15 15 11 19 19 15 13 5 20 8 9 14 7 6 9 19 8 25 ? 49 5 12 12 9 20 9 19 ! !

Method 2: Using a Dictionary Iterable
Using a dictionary is indirectly the same as using two lists. The only difference here is the two lists are merged as one single data structure. Python dictionary allows users to subscript strings to the dictionary name, making element access easier. It is shown in the code below:
charstr='abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
chars=list(charstr)
nums=[str(i) for i in range(1,53)]
orddict=dict(zip(chars,nums))
with open('data.txt','r') as f:
data=f.read()
output=''
for i in range(len(data)):
if data[i] in chars:
output=output+orddict[data[i]]+' '
elif data[i]==' ':
output=output+'\t'
else:
output=output+data[i]+' '
print('Output by Dictionary : '+output)
The lists used in this code snippet are the same as those used in code for converting alphabet letters to numbers using two lists. But here, a dictionary object is created, called orddict, using the zip() function of dict(), which contains the keys as letters from the English alphabet and values as their corresponding numbers. It looks something like this:
{'a': '1', 'b': '2', 'c': '3', 'd': '4', 'e': '5', 'f': '6', 'g': '7', 'h': '8', 'i': '9', 'j': '10', 'k': '11', 'l': '12', 'm': '13', 'n': '14', 'o': '15', 'p': '16', 'q': '17', 'r': '18', 's': '19', 't': '20', 'u': '21', 'v': '22', 'w': '23', 'x': '24', 'y': '25', 'z': '26', 'A': '27', 'B': '28', 'C': '29', 'D': '30', 'E': '31', 'F': '32', 'G': '33', 'H': '34', 'I': '35', 'J': '36', 'K': '37', 'L': '38', 'M': '39', 'N': '40', 'O': '41', 'P': '42', 'Q': '43', 'R': '44', 'S': '45', 'T': '46', 'U': '47', 'V': '48', 'W': '49', 'X': '50', 'Y': '51', 'Z': '52'}
As can be seen in Line 10 of the code, the dictionary can be subscripted using the key value, which in this case is letters of the alphabet. This method shall be preferred over the method using two lists because it reduces the time complexity of the program. It eliminates the need to find the index of letters in the chars list. It produces the same output as above:
Output by Dictionary : 34 5 12 12 15 5 22 5 18 25 15 14 5 , 34 15 23 1 18 5 25 15 21 4 15 9 14 7 ?
38 15 15 11 19 19 15 13 5 20 8 9 14 7 6 9 19 8 25 ? 49 5 12 12 9 20 9 19 ! !
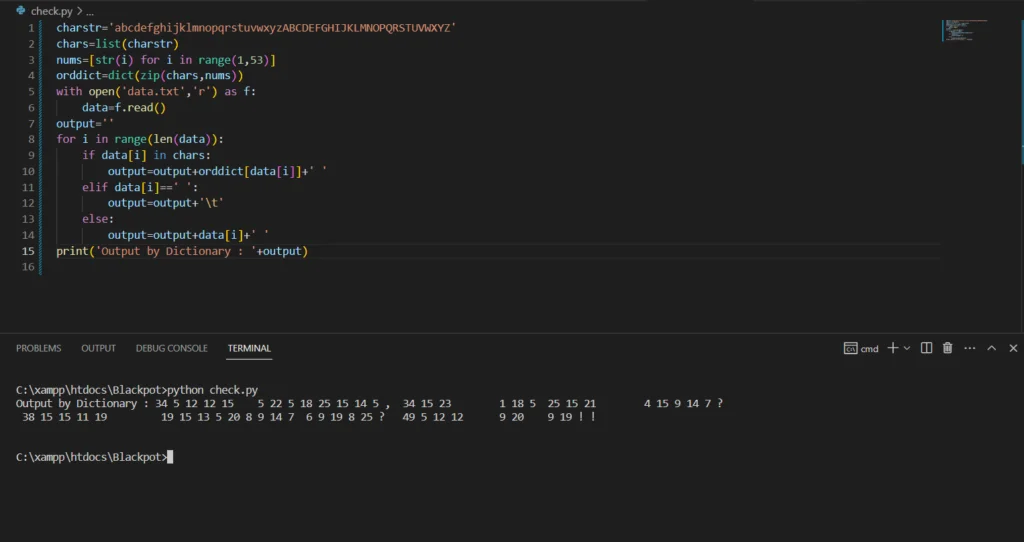
Also read: Python Dictionary (Dict) Tutorial
Method 3: Using Ord() Function of Python
The ord() function of Python returns an integer value, the ordinal number of characters assigned by ASCII. The ordinal number of character ‘A’ is 65. The rest of the characters are ordinal of character before it incremented by 1. So, ‘B’ is 66, ‘C’ is 67, and so on. Similarly, the ordinal number of ‘a’ is 97. So the ordinal number of ‘b’ is 98, ‘c’ is 99, and so on. Now a little math is required to convert these ordinal numbers to numbers assigned in this article.
As ‘a’ is 1, the ordinal number of ‘a’ returned by ord() function shall be subtracted by 96. It returns an output 1 as:
ord('a')=97
required_ord('a')=ord('a')-96=97-96=1
If it is continued on other lowercase letters, the same result is obtained. So, the required ordinal number of ‘b’ can be obtained by subtracting the output of ord(‘b’) by 96
But when it comes to letters in uppercase, the math changes. As uppercase letters are assigned ordinal numbers 65 and onwards, the required ordinal number would be 27 and onwards. The following math is applied:
ord('A')=required_ord('A') + num_to_be_subtracted #Here num_to_be_subtracted is the number which shall be subtracted from value of ord('A') to get required_ord('A')
num_to_be_subtracted=ord('A')-required_ord('A)=65-27=38
This math tells that 38 should be subtracted from the output of ord() function when the letter is an uppercase letter to receive the ordinal number assigned in this article. The following code demonstrates it:
with open('data.txt','r') as f:
data=f.read()
output=''
for i in range(len(data)):
if data[i].isalpha() and data[i].islower():
num=str(ord(data[i])-96)
output=output+num+' '
elif data[i].isalpha() and data[i].isupper():
num=str(ord(data[i])-38)
output=output+num+' '
elif data[i]==' ':
output=output+'\t'
else:
output=output+data[i]+' '
print('Output by ord() function: '+output)
In the above code snippet, there is no need to maintain multiple lists, unlike earlier codes. The isalpha() function of a string tells the program if the character in question is an alphabet letter or not. The islower() function informs the program whether the string in question is lowercase. Similarly, the isupper() function informs whether the string is in upper case or not. In the if block of Lowercase variables, the output of ord() value is subtracted by 96 to receive the corresponding required ordinal number, and the output of ord() function for the uppercase character is subtracted by 38. It produces the same output as above:
Output by ord() function: 34 5 12 12 15 5 22 5 18 25 15 14 5 , 34 15 23 1 18 5 25 15 21 4 15 9 14 7 ?
38 15 15 11 19 19 15 13 5 20 8 9 14 7 6 9 19 8 25 ? 49 5 12 12 9 20 9 19 ! !

Also Read: Python chr() and ord()
Conclusion
Conversion of alphabet letters to numbers is a simple task in python. The data structures provided in Python make the task very easy to implement and execute. Here three methods have been discussed to implement the task. The first method, through two lists, is lengthier and has more time and space complexity than the other two. The second method, through dictionary iterable, is a comparatively shorter method having smaller time and space complexity than using two lists. But using the ord() function of Python provides the shortest and most straightforward method to implement the task. It has a constant time and space complexity, which is O(1). Hence, the ord() function shall be used to convert alphabet letters to numbers
References
https://stackoverflow.com/questions/4528982/convert-alphabet-letters-to-number-in-python