In this article, we will deploy Flask Application onto a Virtual Private Server (VPS) using Apache Webserver Software and mod_wsgi WSGI.
What is VPS?
VPS, which stands for Virtual Private Server, is a virtual machine sold as a service by various hosting companies. You can think of it similar to laptop CPU hardware but in the raw form, i.e., without the Screen, keyboard, etc.
Various companies like Google, Amazon provide Cloud server services (GCP, AWS, etc.). As a part of the service, you can lend various servers situated in different parts of the world. They charge you based on different standards like the hours used, CPU utilization, etc.
Using a Cloud server gives you several advantages like:
- Servers running 24 hrs – With Cloud Servers, your application deployed on them will be running 24hrs. In the case of the localhost(your laptop), you need to keep it running for 24 hrs which is not practical.
- Scalability – In case your storage is full, you can scale out your services(Storage, RAM, etc.) quickly in the cloud.
- More Secure – Since all the hardware is present in the Cloud center, It is more secure.
- Accessibility – You can access your Cloud server from any laptop or computer.
In this tutorial, We will be using the AWS – Amazon Web Services for deployment purpose. You can go with any of the VPS providers.
Software needed to run an application on the internet
Now, a Flask Application, deployed onto a VPS need to perform the following:
- Host Static Files like the HTML/ CSS/ JS
- Handle Http requests
- Recover from Crashes
- Scale-up when required
To perform all these tasks, we require different the following softwares:
- A Web server software – Apache, Nginx, etc
- A Web Server Gateway Interface (WSGI) application server – Mod_WSGI, Gunicorn, etc
- Your Actual Web Application – Written using Flask, Django, etc
Here a web server software like Apache is required to handle the domain logic and also to accept the HTTP requests. The appropriate requests are then sent to the main web Application(Flask) via the WSGI application server (like mod_wsgi, Gunicorn, etc)
The WSGI application server provides the Gateway interface between the Apache software and the Flask application. WSGI is for the frameworks written in python (Django/Flask)
The primary use of WSGI is to convert the requests into a python readable format and send it to the application code (written in Flask-python), which then performs the necessary functions and returns the response Webpage.
Therefore, WSGI works as the gateway between the Apache and the Flask application.
Let us look at them individually
What is Web Server Software?
Web Server software’s primary role is to host websites on the internet. It acts as a middleman between the server and the client machines.
These software applications handle the client requests and then return the physical files(templates/data) present on the server and display them out to the clients.
Examples of Web Server Software are Apache, NGINX etc.
In this article, we will use the good old Apache server, which hosts almost 47% of the web applications on the internet
What is mod_wsgi WSGI Application Server?
Mod_wsgi is an Apache HTTP Server module that provides a WSGI compliant interface for hosting Python-based web applications under Apache. it supports Python versions 2 and 3.
It acts as an interface between Apache software and our Flask application situated on the Cloud server.
Deploying a Flask Project on a VPS
The first step is to select a server from a VPS provider. Any VPS providing companies will have several servers running on different OS systems, as shown

After selecting the required one, you will have to go through some steps to set up and launch the server.
Do note that you have the HTTP port (80) enabled on your Cloud server. Port 80 is the port that the Web server software(Apache, etc.) “listens to” or receives requests from a Web client.
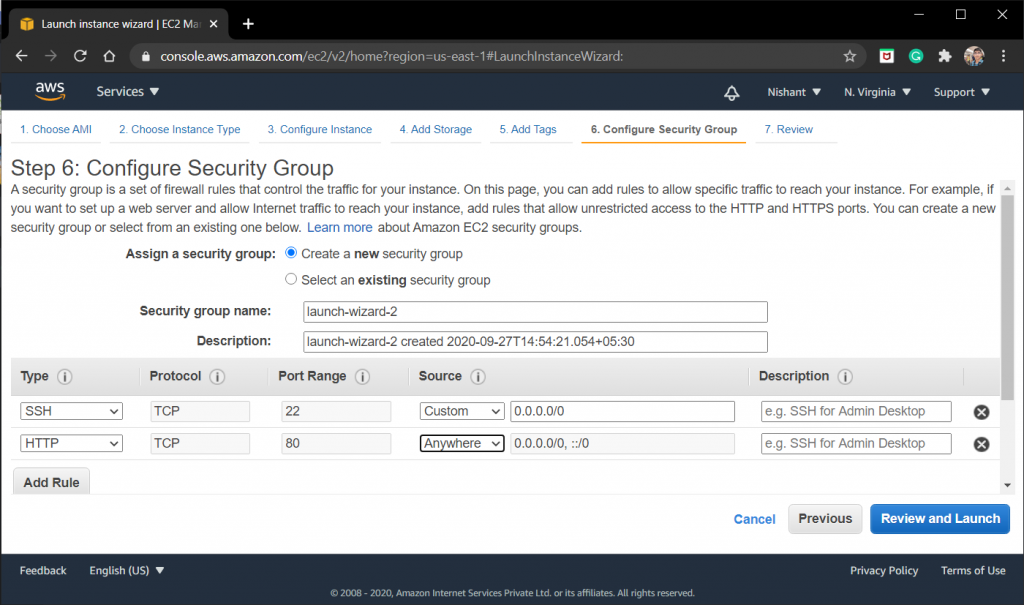
Once the server is set-up, you will get the IP address or the Public DNS of the server, which will be later required to connect to the cloud server.

1. Connecting to the Cloud Server from the Local Computer
We use the ssh command to connect to the server. To connect to the Cloud server from our local computer, run the following commands:
- Using Mac or Linux:
In the shell, simply run the code:
ssh username@<server_IP>
In case you are using an Ubuntu server, the username is ubuntu.
- Using Windows
For windows, we use a software called PuTTY, which you can download from directly from the internet. Once downloaded, enter the cloud_IP in there and hit open.

My cloud_IP/Public DNS which I will be using to access my server is:

For AWS, we need to use an additional private security key as well. Hence depending on the VPS provider, you might also need to add the security_key
Once it is done, you will be logged into the cloud server OS.

2. Installing necessary packages
Once the server is connected, it will be similar to the new ubuntu computer. We first need to install all the important packages into it.
So run the following commands:
Installing Upgrades
sudo apt update
sudo apt upgrade
Always update the system, after starting the server.
Installing apache2 and mod_wsgi on the server
Now let’s first Install Apache2 and the mod_wsgi packages on the system
sudo apt install apache2
sudo apt install libapache2-mod-wsgi
To check whether Apache2 is working or not, go to the Public DNS/IP address of your Cloud server. You will see the Apache Default Page.
Installing python3-pip
Now Install python3 and pip on to the system using:
sudo apt install python-pip
Installing Flask and its extensions
Now install Flask Framework and all other required Flask Extensions like Flask_SQLAlchemy, Flask_login, Flask_wtf, etc
sudo pip install flask
sudo pip install flask_sqlalchemy
....
....
Just install whatever Flask Extensions you require for your Flask Project.
3. Transferring the Flask project from the Local Machine to the Cloud Server
You can create a new directory and code the entire Flask app in there itself using the nano text editor.
mkdir flask_project
cd flask_project
A better option is to code the project on your local machine and then transfer the flask_project folder to the cloud server.
To transfer the file, we use the code
scp -i <path/to/secret_key> -r <path/to/file> ubuntu@<public_DNS>:<path/to/destination>

In AWS, we also need to insert a security key. If your VPS provider does not have any security_key, then remove the key_path from the command
Link the Flask Application Project Directory flask_project present in the Home Directory, with Apache’s Configurations (present in the /var/www/html directory). We do it using the code:
sudo ln -sT ~/flask_project /var/www/html/flask_project
4. Coding the Flask Application
Inside the Flask_project folder, you should be having all the Flask files – app.py, models.py, Templates, etc.
Let us code a simple Flask Application, so in the flask_project directory, create a file app.py
sudo nano app.py
and add the code:
from flask import Flask
app = Flask(__name__)
@app.route('/blogs')
def blogs():
return 'Welcome to The Blog App'
@app.route('/blog/<int:id>')
def blog(id):
return "The Blog id is {}".format(id)
if __name__ == '__main__':
app.run()
After that, we now have to create a WSGI file. For that create a .wsgi file with the same name as the main Flask Application File. In my case, it is app.wsgi
using nano app.wsgi, add the code:
#flaskapp.wsgi
import sys
sys.path.insert(0, '/var/www/html/flask_project')
from app import app as application
Great!! Now we need to enable the mod_wsgi for Apache to interact with the Flask App.
For that go to /etc/apache2/sites-enabled/:
cd /etc/apache2/sites-enabled/
Open the conf file 000-default.conf using nano Text editor and below DocumentRoot /var/www/html line, add the code:
WSGIDaemonProcess flask_project threads=5
WSGIScriptAlias / /var/www/html/flask_project/app.wsgi
<Directory flask_project>
WSGIProcessGroup flask_project
WSGIApplicationGroup %{GLOBAL}
Order deny,allow
Allow from all
</Directory>

Running the Flask Application
That’s it now restart the apache server:
sudo service apache2 restart
And go to the public DNS, the website will be up and running !!

Similarly to the other URL

Perfect!
Conclusion
Your Flask application is up and running on the cloud server. Do Try to Run your own Flask Application on the Cloud Server and let us know what you think. See you guys next time 🙂