When getting started with pandas or even surfing on websites for queries related to pandas operations, we often come across the inplace parameter present in the code. The default value for inplace is set to False.
In this article, we’ll explore the function of inplace parameter when performing operations on Dataframe.
What Does The inplace Parameter Do?
inplace=True
is used depending on if we want to make changes to the original df or not.
Let’s consider the operation of removing rows having NA entries dropped from it. we have a Dataframe (df).
df.dropna(axis='index', how='all', inplace=True)
In Pandas the above code means:
- Pandas create a copy of the original data.
- Performs the required operation on it.
- Assigns the results to the original data. (Important point to consider here).
- Then deletes the copy.
The above code returns nothing but modifies the original Dataframe.
If inplace set to False
then pandas will return a copy of the Dataframe with operations performed on it.
In Pandas we have many functions that has the inplace
parameter.
So, when we do df.dropna(axis='index', how='all', inplace=True)
pandas know we want to change the original Dataframe, therefore it performs required changes on the original Dataframe.
Inplace = True In Action
Let’s see the inplace parameter in action. We’ll perform sorting operation on the IRIS dataset to demonstrate the purpose of inplace
parameter.
You can know more about loading the iris dataset here.
# Importing required libraries
from sklearn.datasets import load_iris
import pandas as pd
#Loading the dataset
data = load_iris(as_frame=True)
df = pd.DataFrame(data.data)
df

Let’s now perform a sorting operation on petal length
feature
df.sort_values('petal length (cm)' , ascending = True) #inplace by default set to False

Now let’s check what happened to our Original dataframe.
df

We just got the original Dataframe when printed even after we applied the sorting operation on it.
So… what just happened?
The above example best demonstrates the application of inplace parameter.
By default, it is set to False and due to this, the operation does not modify the original Dataframe. Instead, it returns a copy on which the operations are performed.
As in the above code, we did not assign the returned Dataframe to any new variable, we did not get a new Dataframe which is sorted.
new_df = df.sort_values('petal length (cm)' , ascending = True , inplace=False)
new_df
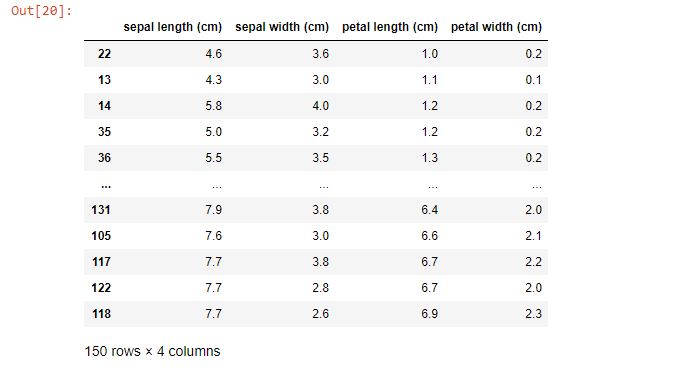
We just assigned the returned Dataframe to a variable we named as new_df.
It is now a sorted copy of the original Dataframe.
An important point to consider here is that the original Dataframe is still the same and did undergo any transformation we specified.
Now let’s see what happens if we set inplace = True
df.sort_values('petal length (cm)' , ascending = True , inplace = True)
Running the code seems to return no output. but wait..!
After checking the original Dataframe we get the essence of what inplace = True
is doing.
df

The original Dataframe got modified after we set inplace=true in Python.
Conclusion
This article was all about the inplace parameter. We now have a certain idea about this sneaky parameter that often sits around in a function without us even realizing it.
As a final thought, we should be very careful while using inplace=True as it modifies the original data frame.
Happy Learning!