The F-string is a formatting technique that allows Python programmers to format strings quickly and easily. By adding the letter ‘f’ as a prefix in the syntax, developers can utilize this popular method for its efficient execution and straightforward syntax. Its convenience surpasses alternatives such as the str. format() method or % formatting. This narrative will explore how to add fixed digits after a decimal with F-strings while highlighting their benefits for string formatting.
The basic use of the f-string method is to format strings according to the user’s choice. Let’s see the example to understand the working of the f-string model.
Syntax Of F-string
f'{value : .number_of_digits f}'
In this syntax, the value contains the number with a decimal point. After ‘.’, we need to define the number of fixed digits we want to print after the decimal point, followed by the ‘f’ letter.
Example of F-string
Value = 'Askpython'
print(f"you can learn Python from {Value}.com")
In this example of f-string, ‘Value’ holds the string we want to use/replace in our printable statement. In the print section, we are printing a string along with the ‘f’ prefix and ‘curly braces’ to represent the exact location of the string to insert in the sentence.

As a result, we can see that the sentence is printed correctly!
How To Add Fixed digits after decimal with f-strings?
Let’s see the example to understand the method of f-string formatting.
Value = 77.45238
print(f'{Value:.3f}')
The Value variable in the above 2-line code holds the float number with a decimal point. There are five digits after the decimal. In the second line of code, we have defined the value of the fixed digit as a 3, so the result should contain only three digits.
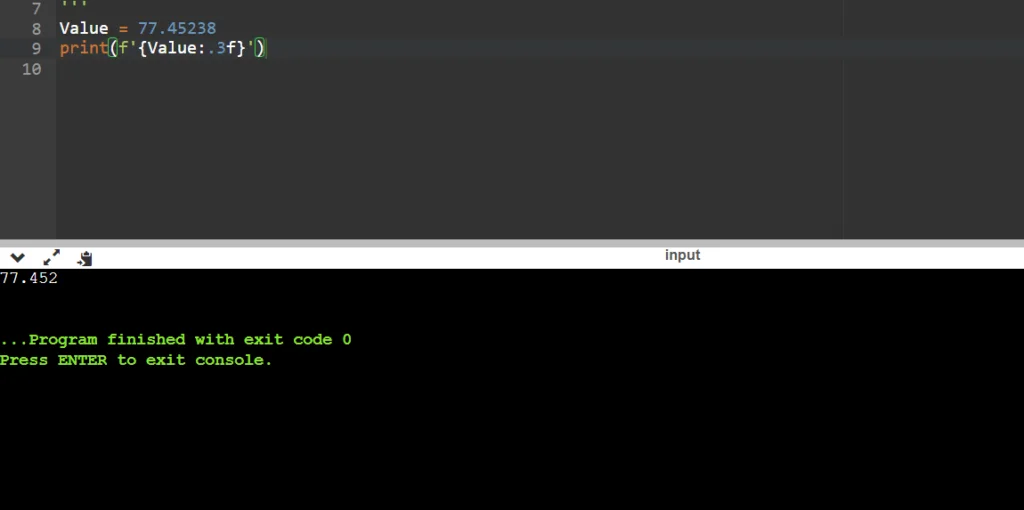
We can see only three digits after the decimal point was printed in the result. So, using the f-string method, we can fix the number of digits printing after the decimal point.
Alternative Methods For Formatting Fixed Decimal Places
Various other methods in Python are used as formatting methods. Let’s see them in detail.
Format() Method
The format() method in Python works similarly to the F-string method. Format() helps to use the formatting technique in Python. The values are inserted with fixed decimal digits in Python.
text = "I got {value:.2f} % marks in exam"
print(text.format(value = 93.2034))
In these two lines of code, the first line represents the simple sentence in which the value is inserted with two precision points. In the second line of the code, the statement is printed with the provided value. This way, we can use the format() method to print the fixed digits in Python.

Round() Function
Technically, the round() function is used to round the numbers after the decimal point. This built-in function in Python helps to ease the task. We need only one line of syntax to execute this function. Let’s see the practical implementation of this round() function using a simple example.
round(Number, Number_of_fixed_digits)
In the syntax of the round() function, the first argument is the floating number, and the second argument is the number of fixed digits that we want after the decimal point. Let’s see the practical example to understand the round() function implementation.
Number = round(7.45, 1)
print(Number)
In this syntax, the Number holds the value and executes the round() function. The number is 7.45. After the application of the round() method, it’ll convert into 7.5.

Comparison of F-string, Format() and Round() Function
F-string, format(), and round() all functions are used for formatting the strings. The format() method and round() function have more complex syntax as compared to the f-string method. Format() and Round() both methods come with the rounding behavior as a default. The format() and round() methods round the number after the decimal digit, but the f-string method helps to fix the digits after decimal points.
Rounding Behavior Of F-string
The f-string method is used for formatting the strings and helps to fix the number of digits after the decimal point. The precision is handled by the F-string method. The %f format is used for rounding float numbers after decimal points. Sometimes, in mathematical calculations, we need float numbers with 2 or 3 precision points but in rounded form. Let’s understand the rounding behavior with %f.
Number = 7.236
print("%.2f" % Number)
In this code, the %f format is used to round the float number. Here the number is rounded up to 2 digits after decimal points.

This rounding behavior of the %f format method can be controlled by the change in syntax. If we write only %f instead of any number, then it will print a float number without rounding behavior.
Advantage Of F-string
F-string is very faster compared to other formatting techniques available in the Python language. The syntax is more understandable and simple to implement. Because of easy to use nature of the f-string method, there is less probability of error while implementing the f-string technique in Python. These features make f-string more useful while formatting strings in Python.
Practical Application Of Fixed Decimal Formatting
Financial Calculation
Fixed decimal counts are very important in the case of financial calculations. There are various sectors like tax calculations, balance sheets, and financial calculations of big companies where calculation and numerical representation of the money is the key. These calculations are in the decimal format. So, to maintain the correct amount or numbers, the rounding system of this money should be perfect. In the case of currency representation, these numbers after the decimal point are also very important while calculating the overall amount. Small errors in these cases may lead to big issues while calculating big amounts and dealing with the percentage values.
For example, different types of loans have different percentage rates, so the decimal values of the numbers also play an important role while calculating the interest amount.
Scientific Calculations
Scientific calculations also contain some areas where decimal formatting and maintaining fixed digits after the decimal point becomes a very important factor in reducing the probability of error. For example, while writing the final measurements as an output. Further calculations with well-rounded measurements become easy to understand. During the graphical representation, the decimal formatting of the numbers helps to represent the data more precisely.
User Interface Design
User interface designs also contain different types of numerical data that should be represented correctly in terms of decimal points. The UI designs may contain a calculator-like structure where maintaining the decimal points are very important to calculate the correct results. Another thing is a data representation of graphs, tables, charts, and any financial calculations where precision and decimal numbers play a very important role in conveying the right numbers and data to the users. Sometimes, we need to fill form fields in terms of percentage. In this case, we need to maintain the formatting and fixed decimal digits after the decimal points.
Summary
In this article, different points related to the formatting of fixed digit numbers after decimal points using f-string are covered. These include basic examples of fixed digits after the decimal point, the f-string method, the rounding behavior of f-string, and other methods to implement the same method to round the numbers after decimal points. Some advantages and practical applications of fixed digits after decimal points are also covered. Hope you will enjoy this article.
References
You can read this for more detail on the f-string method.