Fractions, when converted into decimals, often result in a string of digits after the decimal point. It is not definitive that the count of digits past the decimal points remain a handful. These digits become irrelevant, especially when they consist solely of trailing zeros. In this article, we are going to deep dive into the nuances of trimming off the trailing zeros from the float values using each of the below technique.
Python offers four effective methods to remove trailing zeros from float values: the to_integral() and normalize() functions, the str() and rstrip() functions, a while loop, and the float() function. These techniques provide diverse approaches to clean up your float numbers and eliminate unnecessary trailing zeros.
- Using the to_integral( ) & normalize( ) functions
- Using the str( ) & rstrip( ) functions
- Using the while loop
- Using the float( ) function
Method 1: Leveraging to_integral() and normalize() Functions
Two tools are better than one, doubling our strength in handling trailing zeros. It is true in this case, when we are trying to combine two functions from the decimal library to remove the trailing zeros in the given input.
The to_integral() function is used to check whether the given input number is blessed with a fractional portion or isn’t, whereas the normalize( ) function is used to remove the trailing zeros without any haze.
One shall get things started by importing the decimal library as shown below.
from decimal import Decimal
Then it is time to feed in the input followed by defining a customised function to combine the capabilities of the to_integral( ) and normalize( ) function as shown below.
ip = Decimal('1.7670000')
def remove(n):
return n.quantize(Decimal(1)) if n == n.to_integral() else n.normalize()
Finally let us have a look at the output.


Method 2: Trimming Zeros with str() and rstrip() Functions
This is a tad bit unconventional since it involves the conversion of the input number into a string and then rip off the trailing zeros using the rstrip( ) function. It might seem unconventional, but it gets the job done effectively!
We’ll start with importing the decimal library, followed by declaring the input as shown below.
from decimal import Decimal
ip = Decimal('1.48975480000')
The input is then converted into a string using the str( ) function.
string = str(ip)
The converted output is passed through the rstrip( ) function within which one ought to specify that the entity to be removed is zero, since the default setting is ‘blank space’.
op = string.rstrip('0')
print ("Truncated output:", Decimal(op))

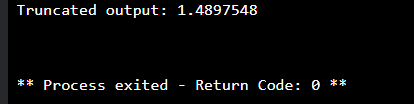
Method 3: Employing a While Loop
A classic while loop can also be very useful for removing the trailing zeros as given below.
ip = "314.509837460000000"
print("Input number:", ip)
while (ip[-1] == '0'):
ip = ip[:-1]
print("Truncated number", ip)
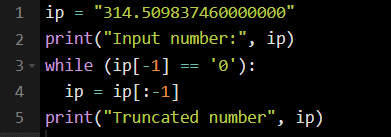
The code is designed to that each trailing zero is being removed one after the other the moment the input is looped through the code.
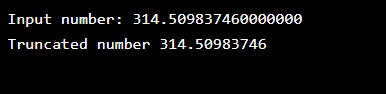
Method 4: Simplifying with the float() Function
The float( ) function will convert the given input into strictly a float value without the space for any trailing zeros. This is a pretty straight forward technique to remove the trailing zeros should there be any in the given input.
ip = "314.509837460000000"
print("Input number:", ip)
op = float(ip)
print("Truncated number:", op)
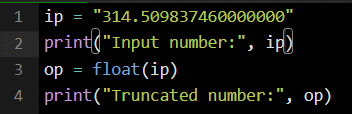

Conclusion:
Now that we have reached the end of this article, we hope this article has provided a clear explanation on the different techniques that can be used to remove the trailing zeros from the floats in Python. Here is another article that details how to use format the contents of MS Excel using to_Excel & Styler functions in Python. There are numerous other enjoyable and equally informative articles in AskPython that might be of great help to those who are looking to level up in Python. To challenge yourself further, can you think of other ways to remove trailing zeros from floats?