Python has four built-in data types data, set, tuple, dictionary, and list. These data types can store multiple collections of data.
A set is an unordered collection of data, it is iterable, mutable, and has no duplicate elements. A tuple is an ordered collection of data but unlike a set, it is not mutable. A dictionary is a collection that contains data in key-value pairs. It is also ordered but unlike a tuple, it is mutable and does not allow duplicates.
On the other hand, a list is a typed array that can store duplicated elements, it is an ordered collection of data, it is dynamic in size and mutable, and its elements can be accessed with their index.
This tutorial will cover all the fundamental things you must know about the list in Python.
Python List
- The list is a mutable sequence.
- We can create it by placing elements inside a square bracket.
- The list elements are separated using a comma.
- We can also create nested lists.
- The list is an ordered collection. So it maintains the order in which elements are added.
- We can access its elements using their index value. It also supports the negative index to refer to the elements from end to start.
- We can unpack its elements to comma-separated variables.
- A List can have duplicate elements. They also allow None.
- It supports two operators: + for concatenation and * for repeating the elements.
- We can slice a list to create another list from its elements.
- We can iterate over list elements using the for loop.
- We can use the “in” operator to check whether an item is present in the list. We can also use the “not in” operator with a list.
- A list is used to store homogeneous elements where we want to add/update/delete elements.
Creating a Python List
A list is created by placing elements inside square brackets, separated by a comma.
fruits_list = ["Apple", "Banana", "Orange"]
We can keep different types of elements in it.
random_list = [1, "A", object(), 10.55, True, (1, 2)]
We can also have nested lists.
nested_list = [1, [2, 3], [4, 5, 6], 7]
We can create an empty list by having no elements inside the square brackets.
empty_list = []
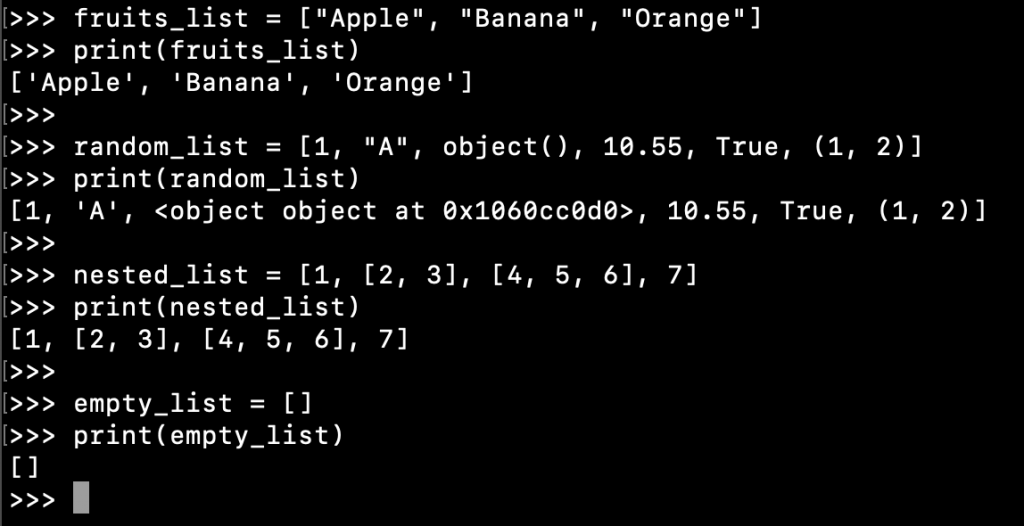
Accessing List Elements
We can access list elements using their index value. The index value starts from 0, i.e, the first element has the index number ‘0’, since it starts counting the index from ‘0’ not ‘1’ so you have to subtract 1 to get the exact element, for example, if you want to access the third element of a list you have to use the index ‘2’.
>>> vowels_list = ["a", "e", "i", "o", "u"]
>>> vowels_list[0]
'a'
>>> vowels_list[4]
'u'
If the index is not in the range, IndexError is raised.
>>> vowels_list[40]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: list index out of range
>>>

We can also pass a negative index value. In this case, the element is returned from the end to the start. The valid index value range is from -1 to -(list length).
This is useful when we want a specific element quickly, such as the last element, second last element, etc.
>>> vowels_list = ["a", "e", "i", "o", "u"]
>>> vowels_list[-1] # last element
'u'
>>> vowels_list[-2] # second last element
'e'
>>> vowels_list[-5]
'a'
Accessing Nested List Elements
We can access nested list elements using nested indexes. Let’s understand this with some simple examples.
nested_list = [1, [2, 3], [4, 5, 6], 7]
# first element in the nested sequence at index 1
print(nested_list[1][0])
# second element in the nested sequence at index 1
print(nested_list[1][1])
# third element in the nested sequence at index 2
print(nested_list[2][2])
The nested element can be any other sequence also that supports index-based access. For example, the result will be the same for the nested list [1, (2, 3), (4, 5, 6), 7].
We can use negative indexes with nested lists too. The above code snippet can be rewritten as follows.
nested_list = [1, (2, 3), (4, 5, 6), 7]
# first element in the nested sequence at third last index
print(nested_list[-3][0])
# last element in the nested sequence at third last index
print(nested_list[-3][-1])
# last element in the nested sequence at second last index
print(nested_list[-2][-1])

Updating a List
We can use the assignment operator to change the list value at the specified index.
>>> my_list = [1, 2, 3]
>>> my_list[1] = 10
>>> my_list
[1, 10, 3]
>>>
Iterating through a List
We can use the for loop to iterate over the elements of a list.
>>> my_list = [1, 2, 3]
>>> for x in my_list:
... print(x)
...
1
2
3
>>>
If you want to iterate over the list elements in the reverse order, you can use reversed() built-in function.
>>> my_list = [1, 2, 3]
>>> for x in reversed(my_list):
... print(x)
...
3
2
1
>>>
Check if an item exists in the list
We can use the “in” operator to check whether an item is present in the list. Similarly, we can also use the “not in” operator with the list.
>>> my_list = [1, 2, 3]
>>>
>>> 1 in my_list
True
>>> 10 in my_list
False
>>> 10 not in my_list
True
>>> 1 not in my_list
False
>>>
Deleting a List
We can use the “del” keyword to delete a list index or the complete list itself.
>>> my_list = [1, 2, 3]
>>> del my_list[1]
>>> my_list
[1, 3]
>>>
>>> del my_list
>>> my_list
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'my_list' is not defined
>>>
Slicing a List
We can use slicing to create a new list from the elements of a list. This is useful in creating a new list from a source list.
The slicing technique contains two indexes separated using a colon. The left index is included and the right index is excluded from the result.
list_numbers = [1, 2, 3, 4, 5, 6, 7, 8]
print(list_numbers[1:3])
print(list_numbers[:4])
print(list_numbers[5:])
print(list_numbers[:-5])

List Concatenation (+ operator)
We can concatenate multiple lists of elements to create a new list using the + operator.
>>> l1 = [1]
>>> l2 = [2, 3]
>>> l3 = [4, "5", (6, 7)]
>>>
>>> l1 + l2 + l3
[1, 2, 3, 4, '5', (6, 7)]
>>>
Repeating List Elements (* operator)
Python List also supports the * operator to create a new list with the elements repeated the specified number of times.
>>> l1 = [1, 2]
>>>
>>> l1 * 3
[1, 2, 1, 2, 1, 2]
>>>
Python List Length
We can get the length or size of the list using the built-in len() function.
>>> list_numbers = [1, 2, 3, 4]
>>> len(list_numbers)
4
The built-in list() Constructor
We can create a list from iterable using the built-in list() constructor. This function accepts an iterable argument, so we can pass String, Tuple, etc.
>>> l1 = list("ABC")
>>> l1
['A', 'B', 'C']
>>>
>>> l1 = list((1, 2, 3))
>>>
>>> l1
[1, 2, 3]
>>>
Python List Functions
Let’s look at some of the functions present in the list object.
1. append(object)
This function is used to append an element to the end of the list.
>>> list_numbers = [1, 2, 3, 4]
>>> list_numbers.append(5)
>>> print(list_numbers)
[1, 2, 3, 4, 5]
2. index(object, start, end)
This function returns the first index of the object in the list. If the object is not found, then ValueError
is raised.
The start and end are optional arguments to specify the index from where to start and end the search of the object.
>>> list_numbers = [1, 2, 1, 2, 1, 2]
>>>
>>> list_numbers.index(1)
0
>>> list_numbers.index(1, 1)
2
>>> list_numbers.index(1, 3, 10)
4
>>> list_numbers.index(10)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: 10 is not in list
>>>
3. count(object)
This function returns the number of occurrences of the object in the list.
>>> list_numbers = [1, 2, 1, 2, 1, 2]
>>> list_numbers.count(2)
3
>>> list_numbers.count(1)
3
4. reverse()
This function reverses the list elements.
>>> list_numbers = [1, 2, 3]
>>> list_numbers.reverse()
>>> print(list_numbers)
[3, 2, 1]
5. clear()
This function removes all the elements from the list.
>>> list_numbers = [1, 2, 5]
>>> list_numbers.clear()
>>> print(list_numbers)
[]
6. copy()
This function returns a shallow copy of the list.
>>> list_items = [1, 2, 3]
>>> tmp_list = list_items.copy()
>>> print(tmp_list)
[1, 2, 3]
7. extend(iterable)
This function appends all the elements from the iterable to the end of this list. Some known iterable in Python are Tuple, List, and String.
>>> list_num = []
>>> list_num.extend([1, 2]) # list iterable argument
>>> print(list_num)
[1, 2]
>>> list_num.extend((3, 4)) # tuple iterable argument
>>> print(list_num)
[1, 2, 3, 4]
>>> list_num.extend("ABC") # string iterable argument
>>> print(list_num)
[1, 2, 3, 4, 'A', 'B', 'C']
>>>
8. insert(index, object)
This method inserts the object just before the given index.
If the index value is greater than the length of the list, the object is added at the end of the list.
If the index value is negative and not in the range, then the object is added at the start of the list.
>>> my_list = [1, 2, 3]
>>>
>>> my_list.insert(1, 'X') # insert just before index 1
>>> print(my_list)
[1, 'X', 2, 3]
>>>
>>> my_list.insert(100, 'Y') # insert at the end of the list
>>> print(my_list)
[1, 'X', 2, 3, 'Y']
>>>
>>> my_list.insert(-100, 'Z') # negative and not in range, so insert at the start
>>> print(my_list)
['Z', 1, 'X', 2, 3, 'Y']
>>> my_list.insert(-2, 'A') # negative and in the range, so insert before second last element
>>> print(my_list)
['Z', 1, 'X', 2, 'A', 3, 'Y']
>>>
9. pop(index)
This function removes the element at the given index and returns it. If the index is not provided, the last element is removed and returned.
This function raises IndexError if the list is empty or the index is out of range.
>>> my_list = [1, 2, 3, 4]
>>>
>>> my_list.pop()
4
>>> my_list
[1, 2, 3]
>>> my_list.pop(1)
2
>>> my_list
[1, 3]
>>> my_list.pop(-1)
3
>>> my_list
[1]
>>> my_list.pop(100)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: pop index out of range
>>>
10. remove(object)
This function removes the first occurrence of the given object from the list. If the object is not found in the list, ValueError is raised.
>>> my_list = [1,2,3,1,2,3]
>>> my_list.remove(2)
>>> my_list
[1, 3, 1, 2, 3]
>>> my_list.remove(20)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: list.remove(x): x not in list
>>>
11. sort(key, reverse)
This function is used to sort the list elements. The list elements must implement __lt__(self, other) function.
We can specify a function name as the key to be used for sorting. This way we can define our own custom function to use for sorting list elements.
The reverse accepts a boolean value. If it’s True, then the list is sorted in the reverse order. The default value of reversed is False and the elements are sorted in the natural order.
>>> list_num = [1, 0, 3, 4, -1, 5, 2]
>>> list_num.sort()
>>> list_num
[-1, 0, 1, 2, 3, 4, 5]
>>> list_num.sort(reverse=True)
>>> list_num
[5, 4, 3, 2, 1, 0, -1]
>>>
List vs Tuple
- The list is a mutable sequence whereas Tuple is immutable.
- The list is preferred to store the same types of data types where we need to add/update them.
- A list requires more memory than Tuple because it supports dynamic length.
- Iterating over a list is slightly more time taking than a Tuple because its elements are not required to be stored in contiguous memory locations.
Conclusion
In this tutorial, we learned that a Python List is a mutable sequence. It is an ordered collection of data, the data in a list can be accessed using its index value. The index value starts with 0 for the very first element in the sequence. The list also supports the negative index to refer to the elements from end to start.
We have also covered Python’s different methods to add, insert, update, and remove elements from a list. Any iterable elements can transform into a list using the Python built-in list() constructor.
Hope this tutorial helps you to understand the concept of List in Python.