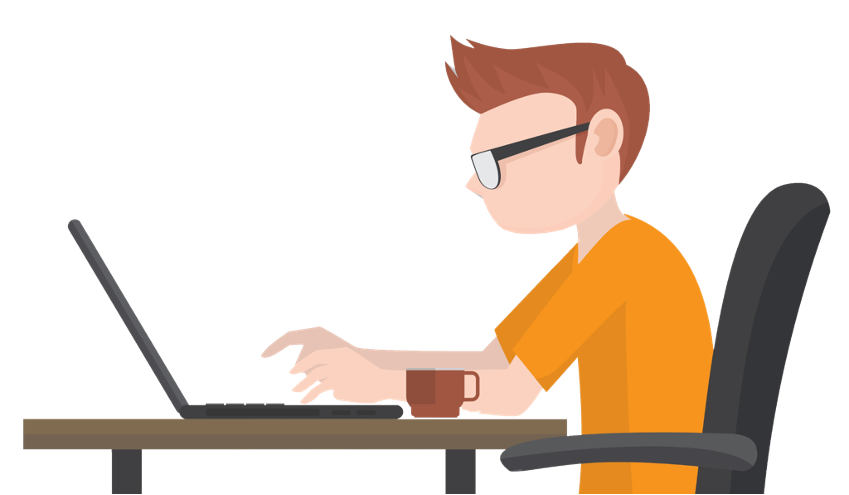
Looking for how to add a new element at the beginning of the list in Python? Go through the complete article.
Python List is a special feature that can hold different kinds of data types there. In a single list, there can be Integers, Strings, Floats, etc can be present altogether. In simple terms, you can think of the list as a big container where different elements can be stored which has the mutable feature. That means, after inserting any element into the list, any expert programmer can do any operation there Like adding, removing, modifying the elements, etc.
From the programmer’s viewpoint, there are infinite times when an element needs to be inserted in the list at a specific position. And if the position is at the very beginning of the list, then the article is only for you. Fortunately, there are four effective methods present to perform such operations.
What is List Prepending in Python?
List Prepending means inserting any element at the beginning of the list. In normal times, when a programmer inserts any element into the list, it saves at the end. But inserting any elements in the beginning means, there is a need to make room for other elements for that new one.
We can take one example to discuss the operation. Suppose, there is a list present as 1, 2, 3, 4 and you need to insert the element 5 at the beginning. It will not be possible with the simple inserting operation that is made for the list data type. We need to use some other approach or other tricks.
We will discuss all of the methods to prepend any element into one list in Python.
List Prepending using Insert() Operation
Insert is one of the inbuilt functions present in the Python program. This Insert operation is highly used for inserting any element into the list. But the same Insert() method can be used for Prepending any Python list as well. We need to use the complete syntax of the method.
There are two arguments present in the Insert operation. The first one is the Index Value & second one will be the value that should be inserted. In the case of the list, the first index value starts with Zero. So, the syntax will always contain zero as the first argument.
Syntax: lit-name.insert(0, value)
series = [10, 20, 30, 40] # List Of Numbers
print("List Before Prepending: ", series) # Printing List
series.insert(0, 50) # Prepending The 50 Element
print("List After Prepending: ", series) # Printing List
In the above code, a list is declared in the first line. Then, the list is printed in the program using the print statement in the second line before performing any operation. Now, the above insert() methods will be used. Here, the number 50 is used for inserting in the beginning.
Now, if again the list is printed, we can see that element 50 has reached the first position. Whereas, all other elements remained at the same spot.

List Prepending using the Slicing Method
Slicing is another great feature present in the Python programming language. Slicing is a process by which we can divide any list or dictionary into two parts. In one part, there might be only one element. And in the second part, the rest of the elements are present.
We will use that approach for Prepending any element into the list of Python. We will use the method to make a new list where the new element will enter first. Later, all the elements of the main list will be entered. The syntax will be like the following:
Syntax: new-list-name = [value] + old-list-name[:]
series1 = [100, 200, 300, 400] # List Of Numbers
print("List Before Prepending: ", series1) # Printing List
series2 = [500] + series1[:] # Prepending The 500 Element
print("List After Prepending: ", series2) # Printing List
In the above code, we can see that a list series1 is declared with some values. We will first print the elements of the series1 list to mark the changes with the new list that will be declared later. Now, another list is declared as the series2 in the third line.
The Second line implies that the element 500 will enter into the list first. Later, all the elements will be entered one by one into the list without any slicing. In this way, the complete new list will be declared and printed in the program.

List Prepending using Plus (+) Operator
The plus operator is a useful tool in high-level languages like Python. Using the plus operator, one can add two integer values. As well as it is used for concatenating any two data types. With the help of the plus operator, the concatenation of one new element can be possible with a list.
Here, the idea is that the new element will first enter into the new list. Then, all the elements of the main list will be entered into the new list. Hence, the Prepending will be done with the concatenation process.
Syntax: new-list-name = [value] + old-list-name
series1 = [100, 20, 300, 40] # List Of Numbers
print("List Before Prepending: ", series1) # Printing List
series2 = [50] + series1 # Prepending The 50 Element
print("List After Prepending: ", series2) # Printing List
In the above program, the list series1 is declared with the values 100, 20, 300, and 40. Now, the list will be directly printed in the program using the Print statement. Now, it is time to declare a new list which will contain all the elements of the first list along with a new one at the beginning.
Here, the new list name is declared as a series2. In the third line, we will follow the syntax structure. We will put the new value number under the Third Braces ([]). It will mark that element as another list with only a single value. Now, the first list will be concatenated with that single-element list. And a new list will form.

List Prepending Using Multiply (*) Operator
The multiply operator can also be useful for Prepending any element into the Python List. However, the implementation process could be simpler compared to any other mentioned method. Here, the element needs to be multiplied a single time to get inserted into the list.
Also, the slicing technique is used in the method to achieve the goal. The idea is to first insert the element into another list and make the list long enough to hold the first declared list. Now, the first list will be merged to the second list using the slicing technique.
Syntax: new-series-name = [value] * (len(old-series-name) + 1)
series1 = ["Example", "Python", "Prepend", "List"] # List Of Numbers
print("List Before Prepending: ", series1) # Printing List
series2 = ["Sample"] * (len(series1) + 1) # Prepending The 'Sample' Element
series2[1:] = series1 # Adding Two Lists
print("List After Prepending: ", series2) # Printing List
In the above code, a list is declared with some string values. We will straightaway print the elements of the list using the print statement in the second line. Now, the syntax will be implemented in the third line. Here, the string ‘Sample’ will be used to Prepend in the list.
Now in the fourth line, the slicing method will be used. The series2[1:] implies that other than the first element, all elements of the old list will be copied to the series2 list. Now, we can easily print the series2 list to check whether the operation is successful or not.

Conclusion
From the above discussion, all the methods effective to put any element into the beginning of the Python list should become clear to you. Most of the programmers and students use the insert method to add any element at any point in the Python list.
But if you find any other method easy & suitable for you, you can go for it. But, first practice the basic ideas of Python programming language which will help you to understand the topic more readily.