- Python if-elif-else statement is used to write a conditional flow code.
- The statements are in the order of if…elif…else.
- The ‘elif’ is short for ‘else if’ statement. It’s shortened to reduce excessive indentation.
- The else and elif statements are optional.
- There can be multiple elif statements.
- We can have only one else block for an if statement.
- The if, else, and elif are reserved keywords in Python.
- Python doesn’t have switch-case statements like other languages. The if-else conditions are sufficient to implement the control flow in the Python scripts.
- We can have nested if-else blocks in Python.
- Python ternary operation allows us to write an if-else statement in a single line. It’s useful when the if-else condition is simple and we want to reduce the code length.
Python if else Syntax
The general syntax if if-else statement in Python is:
if condition:
# code
elif condition:
# code
... # multiple elif statements
else:
# default code
- Every if-elif statement is followed by a condition.
- If the condition evaluates as True, then the code in that block gets executed.
- Once the code in any of the block is executed, the control flow moves out of the if-elif-else block.
- If none of the conditions are True, then the else block code is executed.
Python if-elif-else Example
Let’s say we have a function that accepts a country name and return its capital. We can implement this logic using if-else conditions.
def get_capital(country):
if country == 'India':
return 'New Delhi'
elif country == 'France':
return 'Paris'
elif country == 'UK':
return 'London'
else:
return None

Earlier, we mentioned that elif statement is optional. Let’s look at another simple example where we don’t have elif statement.
def is_positive(num):
if num >= 0:
return 'Positive'
else:
return 'Negative'
Even the else block is optional. Let’s look at another example where we have only if condition.
def process_string(s):
if type(s) is not str:
print('not string')
return
# code to process the input string
In case you are wondering what is with empty return statement, it will return None to the caller.
Python if-else in One Line
Let’s say we have a simple if-else condition like this:
x = 10
if x > 0:
is_positive = True
else:
is_positive = False
We can use Python ternary operation to move the complete if-else block in a single line.
The syntax of ternary operation is:
value_true if condition else value_false
Let’s rewrite the above if-else block in one line.
is_positive = True if x > 0 else False
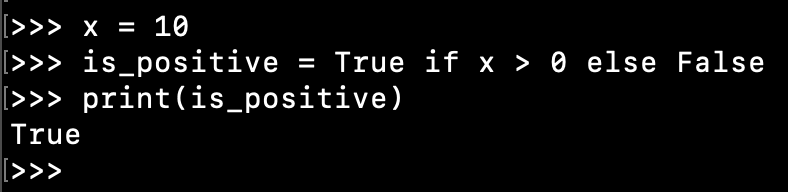
Nested if-else Conditions
We can have multiple nested if-else conditions. Please be careful with the indentation, otherwise the result might be unexpected.
Let’s look at a long example with multiple if-else-elif conditions and nested to create an intelligent number processing script.
# accepting user input
x = input('Please enter an integer:\n')
# convert str to int
x = int(x)
print(f'You entered {x}')
if x > 0:
print("It's a positive number")
if x % 2 == 0:
print("It's also an even number")
if x >= 10:
print("The number has multiple digits")
else:
print("It's an odd number")
elif x == 0:
print("Lovely choice, 0 is the master of all digits.")
else:
print("It's a negative number")
if x % 3 == 0:
print("This number is divided by 3")
if x % 2 == 0:
print("And it's an even number")
else:
print("And it's an odd number")
Here are the sample output from multiple iterations of this code.
Please enter an integer:
10
You entered 10
It's a positive number
It's also an even number
The number has multiple digits
Please enter an integer:
0
You entered 0
Lovely choice, 0 is the master of all digits.
Please enter an integer:
-10
You entered -10
It's a negative number
And it's an even number
Conclusion
Python if-else condition allows us to write conditional logic in our program. The syntax is simple and easy to use. We can use ternary operation to convert simple if-else condition into a single line. Please be careful with the indentation when you have multiple nested if-else conditions.