A module in Python is a Python file (a file with .py extension) that contains some Pythonic code in it. A code being Pythonic means that it will have some meaning for the Python interpreter. A Python script can be written directly on the terminal. But the code written in the terminal is lost when the shell session ends. Python module helps save the code permanently in the system.
A module can be directly interpreted by the Python interpreter or can be imported by another file to work upon. Importing modules brings a lot of convenience to us. Once you’ve created a module, you can even upload it to the Python Package Index and start its distribution. Then through the Python Package Index, anyone in the entire world can install your code into their system just with a single lined pip shell command.
PyPI has many popular libraries that have a pool of functions and methods to help you build your project. Some of those libraries are NumPy, Pandas, Matplotlib, Pygame, Turtle, and a lot more.
pip
is a shell command that you can install with the Python language, or you can separately install it from the official PyPI webpage. It is used to install/uninstall/upgrade any Python module, package, or library available on PyPI from CLI( command line interface). Once you’ve installed it, you can import it into your Python module and use any part of it.
But installing a module separately in the command line can be tedious, and we programmers don’t hate hard work. As programmers, we like to make a process as easily fluent as possible. So in this article, I will teach you how to install a Python module within the code itself and check if a module is installed or not. So let’s get started.
How to install a module using the pip
shell command?
Using the pip command, you can install modules from the PyPI (Python Package Index) repository and see if they are already installed in Python. Initially, make sure pip has been installed on your system. You can install pip from the official PyPI webpage. Running the pip command on the terminal will allow you to verify.
pip
Related: Learn about pip in depth.

Now that you’ve installed pip, you can install any module or package available on PyPI by using the pip install <module-name>
command. Let’s try to install numPy into our system.
To install numPy, type the following command in your terminal.
pip install numpy

Now that we have learned to install a module, we’ll learn how can we find if a module is present in our system or not in the code itself.
How to check if a module is installed in Python?
You can check if a module is present in your system or not directly by the pip
check
command or check manually by pip list
command, which returns a list of all the Python modules present in your system. But that’s too much work. Let’s write a function that will check if a module is installed in Python or not.
There are various ways through which you can check if a module is installed in your system or not. We’re going to check each one of them one by one.
Using import
keyword and try-except
block
try:
import numpy
print("Module is installed.")
except ImportError:
print("Module is not installed")
In the above block of code, use the try-except block to check if we can import a module or if we get an error. If we get an error that means the module hasn’t been installed. So in the except block, we printed that the module is not installed.
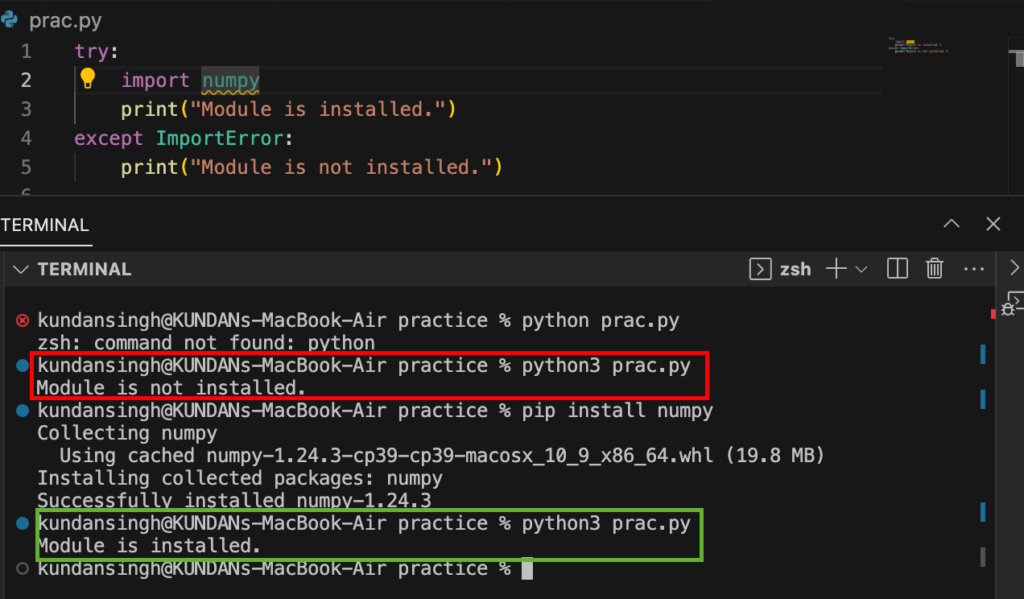
In the output, you can see initially, when the numpy module wasn’t installed, it was saying Module is not installed, and later when we installed it and ran the program again, it said that the module was installed.
Using the sys
module
sys
module is a popular Python module used to perform system-related tasks. It can be used to check whether a module is imported or not. Let’s see how we can do it.
Related: Learn about the sys module in depth.
import sys
import numpy
if 'numpy' in sys.modules:
print("Module is imported.")
else:
print("Module is not imported.")

The sys.modules
is a dictionary that stores all the modules that can be used by the program. To check if a module is imported or not, we’d just have to check if it’s present in the dictionary or not.
Using the pkgutil
module
import pkgutil
module_name = 'numpy'
loader = pkgutil.find_loader(module_name)
if loader:
print("Module is installed.")
else:
print("Module is not installed.")

The find_loader method of the pkgutil module can be used to find a module in the system. If the module is present in the system, it loads the module, or else it will store a none value. So we just have to check if it stores a none value or not.
Now that we’ve learned how to check if a module is present in the system or not let’s see how we can install a module through code.
Creating a function to install a module through code
Now we’re finally going to see how we can install a module through the code itself. To do so, we’ll have to run the shell command within the code. For this purpose, we have various libraries. But the best one among them is the subprocess module. Let’s see how we can install a module using the subprocess module.
import subprocess
def install(module):
subprocess.check_call(['pip', 'install', module])
print(f"The module {module} was installed")
install('numpy')

To run a shell command, we need to use the check_call
method of the subprocess module. This method takes a list as an argument. Our tool is pip
and the command is install
. So we pass a list containing ‘pip’ ‘install’ and the ‘<module-name>’ as the argument. So we just pass the module -name as numpy and run the code and the module is installed.
Application
The process of checking if a module is installed or not is simple and has around 4-5 lines of code only. It’s a good practice to always write code that minimizes the chances of errors. Always checking if a module is installed or not before importing minimizes the chances of any errors. So we can just create a function that first checks if a module is installed or not, and if it’s not installed, it will install it and then import it. To do so we just need to combine the two functions we created in this article.
Error handling while importing or installing files
The most common error that can occur while importing a module is a Module Not Found Error. It can be due to spelling errors, trying to import an uninstalled module, or there even maybe system issues. Whenever you encounter an error, there’s likely a spelling error. So you must always look for spelling errors.
Conclusion
Checking if a module is installed or not and installing it within the code not only makes the work easy but also minimizes the chances of any potential errors. So you must always include it while building any kind of project. Finding ways to minimize the chances of errors prevents a lot of waste of time and energy.
References
Official Python Documentation.
Stack Overflow thread for the same question.